Tutorial: Physics in a 2D platformer game
It's time to make our player character be able to actually land on the floor without falling through it. Doing so is fairly straightforward and sets up a basis for the steps to come.
Making floors solid
First we need to determine where each edge of the player's bounding box is. In order to do so, we will define 4 points on the bounding box edges to check for collisions with.
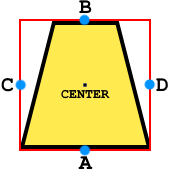
To handle everything related to world collisions, we're going to add a method called handleCollisions() to our PhysicsObject class and call it from our Update method (remember to first do all movement changes and then check to resolve collisions).
public virtual void Update(GameTime gameTime) { position.Y += (float)(GRAVITY * gameTime.ElapsedGameTime.TotalSeconds); handleCollisions(); } private void handleCollisions() { //bottom if (Level.isSolid(getTileCenterX(), getTileBottom())) { position.Y = (getTileBottom() - 1) * Level.TILE_SIZE; } }
In the code above, you'll see how we make use of the getTileCenterX() and getTileBottom() methods we wrote for our BBox class. Point A is located at the center and bottom of our bounding box, so we use those coordinates to retrieve the tile column and row numbers. Those numbers are fed into the isSolid method which checks if the value in the array at the specified indexes is a 1 (solid) or a 0 (non-solid).
If it turns out point A is inside a solid tile, we reposition the player character so that it sits right above this tile and no longer overlaps it.
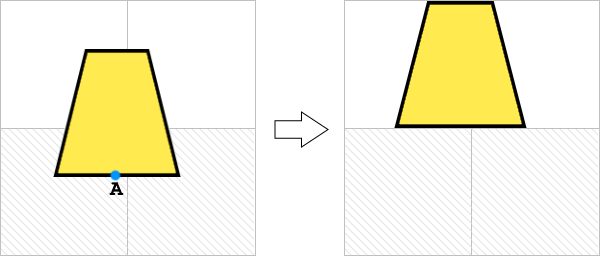
A quick side-note about the isSolid() method: if you're implementing this yourself and using the level array defined on the first page, do take note that your X's and Y's aren't flipped around incorrectly. The level array was defined in such a way that when you look at it, you basically see the level layout as it'll end up in the game. I did this to make it easier to edit the array and know what the level will end up looking like. This does mean, however, that to get the correct data from this array you'll need to access the tile data by doing Level.data[y,x] instead of Level.data[x,y].
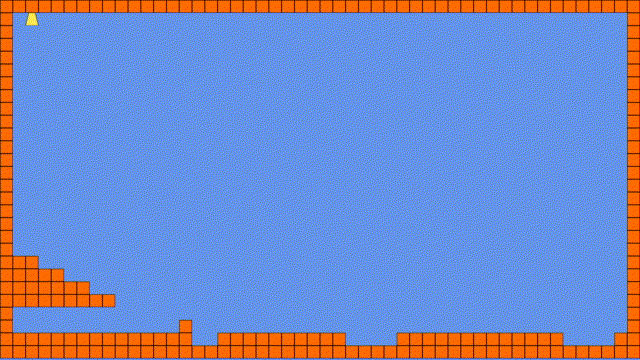
Yay! Solid ground. We're not there yet, though, we need to make sure you can't move through walls either. Also, we might want to implement some form of jumping in the future, and we don't want the player to jump through ceilings. All in all, there's more work to be done!